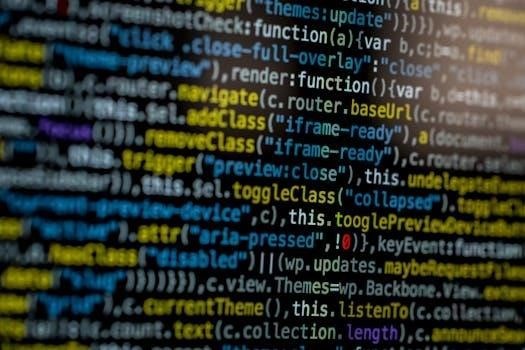
JavaScript Tutorial PDF⁚ A Comprehensive Guide
This free handbook provides a comprehensive guide to JavaScript․ It covers everything from the basics to advanced techniques, offering a foundation for building web applications․ You can also find paid versions for added support and more in-depth learning․ Downloadable PDF versions are available․
JavaScript, often abbreviated as JS, is a high-level, dynamic, weakly typed, prototype-based, multi-paradigm, and interpreted programming language․ It’s a cornerstone technology of the World Wide Web, enabling interactive and dynamic content on websites․ Initially designed for client-side scripting, JavaScript has expanded its reach to server-side development, mobile applications, and even game development․ Its versatility and widespread adoption make it an essential skill for any aspiring web developer․ This tutorial will guide you through the core concepts of JavaScript, providing both theoretical insights and practical examples to help you grasp its functionalities․ We’ll explore how it manipulates the Document Object Model (DOM), handles user events, and works with asynchronous operations․ The introduction will also touch upon the significance of JavaScript in the modern web development landscape and its integration with other technologies․ By the end of this section, you’ll have a solid understanding of what JavaScript is and its role in creating interactive web experiences․ Get ready to dive into the world of JavaScript!
Why Learn JavaScript?
Learning JavaScript is crucial for anyone looking to pursue a career in web development, and for good reason․ It is the language of the web, powering interactivity and dynamic content on virtually every website․ With JavaScript, you can create responsive user interfaces, handle user inputs, and fetch data from servers, making websites engaging and functional․ Beyond web development, JavaScript has expanded into areas like mobile app development with frameworks like React Native, server-side programming with Node․js, and even game development․ This versatility means that mastering JavaScript opens doors to numerous opportunities․ Furthermore, the JavaScript community is vast and active, providing abundant resources, libraries, and frameworks to learn from․ Learning JavaScript empowers you to create a wide range of applications, from simple web pages to complex, single-page applications․ This tutorial will help you grasp the fundamentals and prepare you for advanced concepts, enabling you to make the most of this powerful language․ Investing your time in learning JavaScript is a smart move with great potential rewards․
Free JavaScript Tutorial Resources
Numerous free resources are available for learning JavaScript, making it accessible for everyone․ This webpage offers a comprehensive free JavaScript tutorial, which is a great starting point․ For a more structured approach, freeCodeCamp․org provides an excellent JavaScript curriculum with hands-on projects․ Many other online platforms like MDN Web Docs offer detailed documentation and tutorials․ YouTube is a treasure trove of free video tutorials, including full courses for beginners․ These resources often come with practical examples, exercises, and projects to reinforce your learning․ You can also find free eBooks covering various aspects of JavaScript․ Look for resources that provide a mix of theoretical explanations and practical coding exercises․ The availability of free resources means that learning JavaScript does not require expensive courses or textbooks․ These resources are designed to help you progress from beginner to advanced levels at your own pace․ Be sure to explore different options to find resources that suit your learning style․ A combination of these free resources will equip you with the skills needed to master JavaScript․
JavaScript Basics⁚ Conditions, Loops, Functions
Understanding conditions, loops, and functions is fundamental to mastering JavaScript․ Conditions, like `if` statements, allow you to execute different code blocks based on whether a condition is true or false․ Loops, such as `for` and `while` loops, enable you to repeat a block of code multiple times, which is essential for iterating through data․ Functions are reusable blocks of code that perform specific tasks, enhancing code organization and efficiency․ These three concepts are building blocks for more complex JavaScript programs․ Learning to use them effectively is crucial for creating interactive and dynamic web pages․ You will start to write basic programs to control the flow of your code․ Mastering these will make it easier to handle more complex logic․ For example, using a loop to iterate over an array and a function to perform actions on each item․ Combining these concepts allows for the creation of powerful and versatile JavaScript applications․ This knowledge provides the foundation for all types of JavaScript development․
Advanced JavaScript Concepts⁚ Objects, Arrays, ES6
Delving into advanced JavaScript concepts involves understanding objects, arrays, and the features introduced in ES6․ Objects in JavaScript are collections of key-value pairs, allowing you to model real-world entities․ Arrays are ordered lists of values, which are used to store collections of data․ ES6 (ECMAScript 2015) brought significant enhancements to JavaScript, including arrow functions, classes, destructuring, and more․ Arrow functions provide a concise syntax for writing functions, while classes offer a way to create object-oriented code․ Destructuring allows you to extract values from objects and arrays easily․ These concepts enable you to write cleaner, more maintainable, and efficient code․ Grasping these topics will significantly improve your ability to handle more complex programming tasks․ This includes using object-oriented principles and taking advantage of modern JavaScript features for better code structure and readability․ They are essential for any serious JavaScript developer, enabling the creation of advanced interactive features and more robust applications․ Understanding ES6 syntax is critical for working with modern JavaScript․
Free JavaScript Books and Ebooks
Numerous free JavaScript books and ebooks are available online, offering valuable resources for learning and mastering the language․ These resources cover a wide range of topics, from fundamental concepts to advanced techniques․ You can find books focusing on ECMAScript specifications, jQuery, and modern JavaScript practices․ Many ebooks are available in formats like PDF, MOBI, and EPUB, making them accessible on various devices․ These free resources are perfect for both beginners looking to start their JavaScript journey and experienced developers seeking to deepen their knowledge․ They often include practical examples, in-depth explanations, and exercises to help you solidify your understanding․ Explore various platforms that provide free access to these educational materials, enabling you to learn at your own pace․ These books and ebooks can help you explore advanced topics such as design patterns, neural networks, and robotics․ Take advantage of these cost-free opportunities to enhance your skills and stay updated with the latest developments in the JavaScript world․ Accessing these resources is a great way to expand your expertise and become a proficient JavaScript developer․
Pro JavaScript Techniques
Exploring pro JavaScript techniques involves delving into advanced concepts and practices that enhance code efficiency, maintainability, and performance․ This includes mastering complex topics like closures, prototypes, and advanced object-oriented programming principles within JavaScript․ Understanding asynchronous programming with promises and async/await is crucial for handling operations efficiently․ Pro techniques also cover optimizing code for speed and memory, employing design patterns, and writing modular, testable code․ Utilizing advanced debugging tools and techniques becomes essential for identifying and resolving complex issues․ Learning how to leverage the latest ECMAScript features ensures you write modern and efficient JavaScript․ Working with higher-order functions, functional programming paradigms, and advanced DOM manipulation techniques are also part of mastering pro-level skills․ Furthermore, understanding how to integrate JavaScript with other technologies and frameworks is a key skill for professional developers․ This involves topics like server-side JavaScript with Node․js, as well as how to interact with databases and APIs․ Focusing on these techniques will elevate your JavaScript skills to the next level․
JavaScript for Beginners⁚ A Step-by-Step Guide
This step-by-step guide offers a structured approach for beginners to learn JavaScript․ Starting with the fundamental concepts, it introduces variables, data types, and operators․ You’ll then progress to understanding control flow, including conditional statements and loops․ The guide covers functions, explaining how to create and use reusable blocks of code․ Next, you will learn about working with arrays and objects, which are essential for handling collections of data․ A step-by-step approach ensures that beginners grasp each topic before moving to the next․ This guide uses clear explanations and practical examples to illustrate concepts․ It focuses on building a solid foundation, making it easier to tackle more advanced topics later on․ Beginners will also learn how to interact with the browser using the DOM (Document Object Model)․ This step-by-step approach is designed to help you gradually build your skills․ By the end of this guide, you’ll be able to write basic JavaScript programs and understand the fundamentals of web development․ This guide includes exercises and quizzes to reinforce learning․
Practical JavaScript Examples
This section provides practical JavaScript examples to demonstrate real-world application․ Examples include creating interactive web elements, such as image hover effects and dynamic content updates․ You will see how to manipulate the DOM to change page elements in response to user actions․ We provide examples of using JavaScript to validate form inputs, ensuring data integrity․ You can learn how to create simple games and interactive elements using JavaScript․ The guide also includes examples of using AJAX to fetch data from servers without page reloads․ You will find examples of working with JSON data, which is common in web applications․ The practical examples also show how to implement event handling for user interactions․ Each example is designed to be clear, concise, and easy to understand, making it easier to apply them in your own projects․ These examples cover a range of scenarios, providing a solid understanding of JavaScript usage․ You’ll find examples of creating carousels and slide shows and implementing basic animations․ By working through these practical examples, you’ll be able to build more interactive and dynamic web pages․
TypeScript⁚ Extending JavaScript
TypeScript is a powerful language that extends JavaScript by adding static typing․ This feature helps catch errors during development, improving code reliability․ The tutorial covers how TypeScript enhances JavaScript with classes and interfaces, enabling better code organization․ It explains the benefits of using TypeScript, especially in large and complex projects․ You’ll learn about TypeScript’s type annotations and how they improve code readability and maintainability․ The guide explores how TypeScript compiles to JavaScript, making it compatible with all browsers․ It includes examples of using TypeScript features like generics and enums for more robust coding․ It also explains how to migrate existing JavaScript code to TypeScript․ This section dives into the benefits of using TypeScript for large projects and for teams, highlighting the advantages of static analysis․ TypeScript makes your JavaScript code more reliable by catching errors early․ It allows you to create more modular and reusable code by leveraging OOP concepts․ The guide shows you practical examples of how TypeScript can be applied to real-world JavaScript projects․ Furthermore, it highlights TypeScript’s compatibility with JavaScript libraries and frameworks․ This introduction to TypeScript will prepare you to utilize its unique advantages․
Modern JavaScript Features
This section explores the latest features of modern JavaScript, including those introduced in ECMAScript 6 (ES6) and beyond․ The tutorial covers arrow functions, providing a more concise syntax for writing functions․ You’ll learn about template literals, which allow for easier string interpolation․ The guide introduces destructuring, a convenient way to extract values from arrays and objects․ It explains the spread and rest operators, which are useful for managing function arguments and array manipulation․ You’ll explore the use of classes for object-oriented programming․ The concept of promises and async/await is covered for handling asynchronous operations more effectively․ The section dives into modules, which help with code organization and reusability․ It details how to use let and const for variable declarations, offering better scope management than var․ The tutorial highlights the importance of these modern features for writing efficient and readable code․ You’ll learn about enhanced object literals for more expressive object creation․ This section includes practical examples demonstrating how to integrate these modern features into your projects․ By understanding these concepts, you’ll be able to write cleaner and more maintainable JavaScript code․ This will allow you to take full advantage of the power and flexibility of modern JavaScript․
JavaScript Libraries and Frameworks
This section delves into the world of JavaScript libraries and frameworks, essential tools for building complex web applications․ It introduces popular libraries like jQuery, which simplifies DOM manipulation and AJAX interactions․ The tutorial explores front-end frameworks like React, a component-based library for building user interfaces, and Angular, a comprehensive framework for large-scale applications․ It covers Vue․js, a progressive framework for building single-page applications and interactive components․ This section also touches on Node․js, a runtime environment that allows you to run JavaScript on the server-side, expanding the possibilities beyond the browser․ You’ll learn about Express․js, a popular framework for building web servers with Node․js․ The guide will cover how to use libraries and frameworks to streamline your development process and create more efficient and scalable applications․ It will highlight the advantages of using these tools, such as code reusability and improved development speed․ You’ll understand how to choose the right library or framework for your specific project needs․ The section will provide practical examples of integrating libraries and frameworks into projects․ By exploring these tools, you will gain a deeper understanding of how to create sophisticated and dynamic web applications․